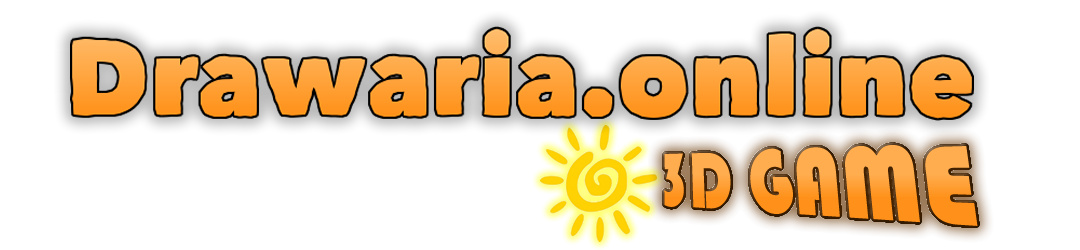
Drawaria online 3D Game
The code you provided sets up a basic 3D platform scene using Three.js, but it doesn't include any interactive gameplay mechanics. To turn this scene into a playable game, you would need to add additional functionality. Here's a basic outline of how you might extend this scene into a simple game and how a user could interact with it:
### Steps to Make the Scene Interactive
1. **Add a Player Character**:
- Create a mesh to represent the player, similar to how the NPCs are created.
- Position the player at a starting location on the terrain.
2. **Implement Player Movement**:
- Use keyboard inputs to control the player's movement. For example, use the arrow keys or WASD keys to move the player forward, backward, left, and right.
- Update the player's position in the `animate` function based on user input.
3. **Collision Detection**:
- Implement basic collision detection to prevent the player from moving through objects like mountains or NPCs.
- You can use bounding boxes or raycasting to detect collisions.
4. **Game Objectives**:
- Define objectives, such as collecting items or reaching a goal area.
- Add visual indicators for these objectives in the scene.
5. **User Interface**:
- Display information like score, health, or time remaining on the screen using HTML elements overlaid on the canvas.
### Example of Adding Basic Player Movement
Here's a simple example of how you might add basic player movement to the existing scene:
```javascript
// Player character
const playerGeometry = new THREE.BoxGeometry(5, 10, 5);
const playerMaterial = new THREE.MeshLambertMaterial({ color: 0x0000ff });
const player = new THREE.Mesh(playerGeometry, playerMaterial);
player.position.set(0, 5, 0);
scene.add(player);
// Player movement controls
const keys = {};
window.addEventListener("keydown", (e) => {
keys[e.key] = true;
});
window.addEventListener("keyup", (e) => {
keys[e.key] = false;
});
function updatePlayerPosition() {
if (keys["ArrowUp"] || keys["w"]) player.position.z -= 0.5;
if (keys["ArrowDown"] || keys["s"]) player.position.z += 0.5;
if (keys["ArrowLeft"] || keys["a"]) player.position.x -= 0.5;
if (keys["ArrowRight"] || keys["d"]) player.position.x += 0.5;
}
// Main render loop
function animate() {
requestAnimationFrame(animate);
updatePlayerPosition();
renderer.render(scene, camera);
}
animate();
```
### How to Play
1. **Movement**: Use the arrow keys or WASD keys to move the player character around the scene.
2. **Objectives**: Depending on the game objectives you implement, the player might need to collect items, reach certain areas, or interact with NPCs.
3. **Avoid Obstacles**: The player should avoid colliding with mountains or other obstacles.
By extending the scene with these interactive elements, you can create a simple 3D platform game that users can play in their web browser.
Published | 20 days ago |
Status | Released |
Platforms | HTML5 |
Author | YouTubeDrawariaGames |
Genre | Simulation |
Tags | AI Generated |
Development log
- enjoy this game20 days ago
Comments
Log in with itch.io to leave a comment.
enjoy this game lol :|